UniformItemsLayout in MAUI Community Toolkit is a layout where all rows and columns will have the same size. UniformItemsLayout is similar to Grid. For positioning the child in a particular cell we no need to specify row and column index in UniformItemsLayout like in Grid, It will automatically positioned child in row and column.
In this article, I’m going to show you how to use UniformItemsLayout in MAUI Community Toolkit.
UniformItemsLayout in MAUI Community Toolkit allow us to limit the maximum number of columns and rows using MaxRows and MaxColumns properties.
MaxRows – Used for Getting/Setting the maximum number of items in a row. MaxColumns – Used for Getting/Setting the maximum number of items in a column. |
Let’s Start
In this sample demo we will use CommunityToolkit.Maui nuget package.
CommunityToolkit.Maui
CommunityToolkit.Maui is a collection of Converters, Behaviours, Animations, Custom Views, Effects, Helpers etc. It simplifies the developers task when building Android, iOS, macOS and WinUI applications using MAUI.
1 – Install CommunityToolkit.Maui nuget package
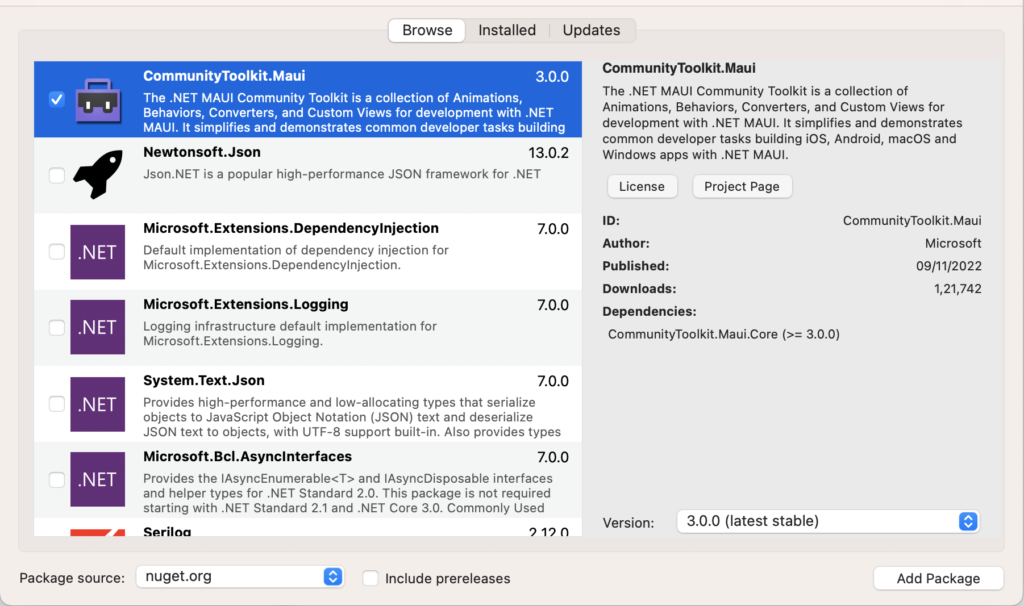
2 – In order to use the .NET MAUI Community Toolkit you need to call the extension method in your MauiProgram.cs file as follows:
using CommunityToolkit.Maui; using Microsoft.Extensions.Logging; namespace MAUIUniformItemsLayoutSample; public static class MauiProgram { public static MauiApp CreateMauiApp() { var builder = MauiApp.CreateBuilder(); builder .UseMauiApp<App>() .UseMauiCommunityToolkit() .ConfigureFonts(fonts => { fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular"); fonts.AddFont("OpenSans-Semibold.ttf", "OpenSansSemibold"); }); #if DEBUG builder.Logging.AddDebug(); #endif return builder.Build(); } }
3 – Setting up the UI
3.1 – Create a MainPage.xaml
<?xml version="1.0" encoding="utf-8" ?> <ContentPage x:Class="MAUIUniformItemsLayoutSample.MainPage" xmlns="http://schemas.microsoft.com/dotnet/2021/maui" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" xmlns:toolkit="http://schemas.microsoft.com/dotnet/2022/maui/toolkit"> <ContentPage.Resources> <ResourceDictionary> <Style TargetType="Button"> <Setter Property="CornerRadius" Value="0" /> <Setter Property="TextColor" Value="Black" /> </Style> <Style TargetType="Label"> <Setter Property="TextColor" Value="Black" /> <Setter Property="FontAttributes" Value="Bold" /> <Setter Property="Margin" Value="0,10,0,0" /> </Style> </ResourceDictionary> </ContentPage.Resources> <ScrollView> <VerticalStackLayout Padding="10" Spacing="15"> <Label Text="UniformItemsLayout with default settings" /> <toolkit:UniformItemsLayout x:Name="UniformItemsLayout_Default"> <Button BackgroundColor="#F5F5DC" Text="Button 1" /> <Button BackgroundColor="#FAEBD7" Text="Button 2" /> <Button BackgroundColor="#FFF0F5" Text="Button 3" /> <Button BackgroundColor="#FFE4E1" Text="Button 4" /> </toolkit:UniformItemsLayout> <Label Text="UniformItemsLayout with MaxColumns=2 and MaxRows=2" /> <toolkit:UniformItemsLayout x:Name="UniformItemsLayout_MaxRows2MaxColumns2" MaxColumns="2" MaxRows="2"> <Button BackgroundColor="#E6E6FA" Text="Button 1" /> <Button BackgroundColor="#D8BFD8" Text="Button 2" /> <Button BackgroundColor="#DDA0DD" Text="Button 3" /> <Button BackgroundColor="#EE82EE" Text="Button 4" /> </toolkit:UniformItemsLayout> <Label Text="UniformItemsLayout with MaxRows=1" /> <toolkit:UniformItemsLayout x:Name="UniformItemsLayout_MaxRows1" MaxRows="1"> <Button BackgroundColor="#FFDEAD" Text="Button 1" /> <Button BackgroundColor="#D2B48C" Text="Button 2" /> <Button BackgroundColor="#F4A460" Text="Button 3" /> <Button BackgroundColor="#B8860B" Text="Button 4" /> </toolkit:UniformItemsLayout> <Label Text="UniformItemsLayout with MaxColumns=1" /> <toolkit:UniformItemsLayout x:Name="UniformItemsLayout_MaxColumns1" MaxColumns="1"> <Button BackgroundColor="#D3D3D3" Text="Button 1" /> <Button BackgroundColor="#A9A9A9" Text="Button 2" /> <Button BackgroundColor="#808080" Text="Button 3" /> <Button BackgroundColor="#696969" Text="Button 4" /> </toolkit:UniformItemsLayout> </VerticalStackLayout> </ScrollView> </ContentPage>
As in the above MainPage.xaml i have used four UniformItemsLayout :
- UniformItemsLayout with default settings : Here there is no Column and Row limit set so based on numbers of children MaxColumns and MaxRows will set.
- UniformItemsLayout with MaxColumns=2 and MaxRows=2 : Here only 4 children will display because MaxColumns is set to 2 and MaxRows is set to 2.
- UniformItemsLayout with MaxRows=1 : All children will arrange in 1 row.
- UniformItemsLayout with MaxColumns=1 : All children will arrange in 1 column.
3.2 – MainPage.xaml.cs
namespace MAUIUniformItemsLayoutSample; public partial class MainPage : ContentPage { public MainPage() { InitializeComponent(); } }
Now, We have completed the coding, Let’s try to run the application.
4 – Result
That’s all for now!
You can check the full source code here.
Happy Coding! 😀
You may also like