The traditional way to implement MVVM (Model-View-ViewModel) using INotifyPropertyChanged interface but now we have a ObservableObject that provides a base implementation for INotifyPropertyChanged and INotifyPropertyChanging and this is exposing the PropertyChanged and PropertyChanging events. So In this article, I’m going to show you How to use ObservableObject in MAUI for Data Binding.
Note : To use ObservableObject we must mark the viewmodel class as partial. |
Let’s Start
In this article you will see :
- What is MVVM?
- Main features of ObservableObject?
- What is ObservableProperty attribute?
- What is RelayCommand attribute?
- How to invoke command when clicking on collectionview item?
1 – What is MVVM?
- It stands for Model View ViewModel.
- It enables the separation between View, Model and ViewModel.
- View – It indicates the user interface where a user can interact with. (eg : xaml)
- Model – the underlying data.
- ViewModel – Intermediary between the View and the Model.
- View and ViewModel are connected using BindingContext.
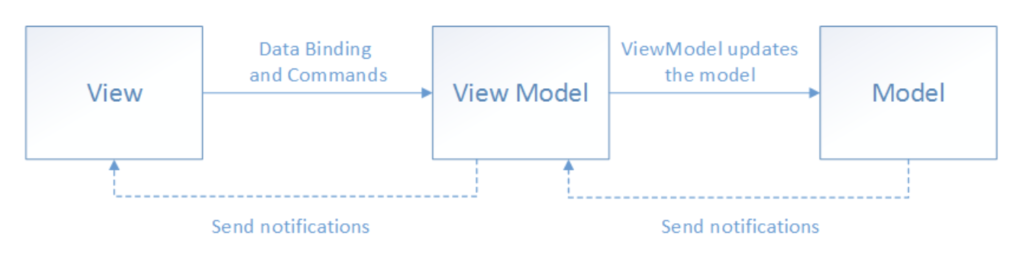
2 – Main features of ObservableObject?
- It provides the base implementation for INotifyPropertyChanged and INotifyPropertyChanging.
- It exposes the PropertyChanged and PropertyChanging events.
- It provides the series of SetProperty() methods that can be used for setting the property value.
3 – What is ObservableProperty attribute?
- It allows us to generate observable properties from annotated fields.
- It reduces boilerplate code.
Without ObservableProperty attribute :
private string _user; public string User { get => _user; set { _user = value; OnPropertyChanged(); } }
With ObservableProperty attribute :
[ObservableProperty] private string user;
4 – What is RelayCommand attribute?
- It allows us to generate relay command property for annotated methods.
- It reduces boilerplate code.
Without RelayCommand attribute :
public class MainPageViewModel : INotifyPropertyChanged { public ICommand AddUserCommand { get; set; } public ICommand DeleteUserCommand { get; set; } public MainPageViewModel() { AddUserCommand = new Command(() => { }); DeleteUserCommand = new Command<string>((user) => { }); } }
With RelayCommand attribute :
public partial class MainPageViewModel : ObservableObject { public MainPageViewModel() { } [RelayCommand] public void AddUser() { } [RelayCommand] public void DeleteUser(string user) { } }
I have created a sample demo project to understand better. So let’s deep dive into this.
In this sample demo i have used CommunityToolkit.Mvvm nuget package.
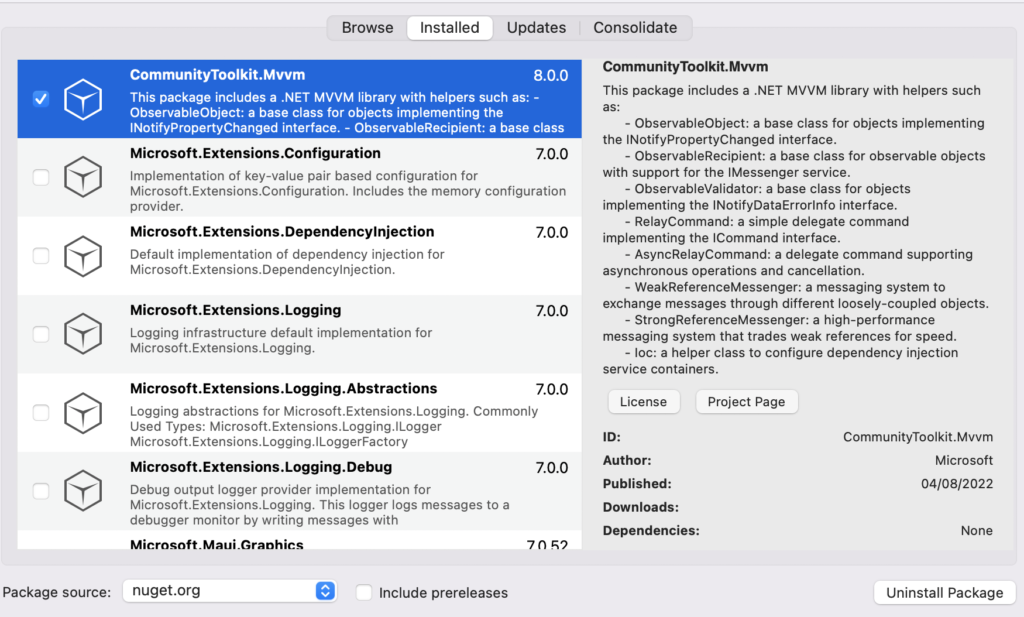
Create a MainPage.xaml
<?xml version="1.0" encoding="utf-8" ?> <ContentPage x:Class="MAUIInvokeCommandOnListItemClickDemo.MainPage" xmlns="http://schemas.microsoft.com/dotnet/2021/maui" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" x:Name="MainPageContainer"> <Grid Padding="30,5" HorizontalOptions="Fill" RowDefinitions="*,Auto" RowSpacing="10" VerticalOptions="Fill"> <CollectionView Grid.Row="0" Margin="0,30,0,0" ItemsSource="{Binding UserList}" SelectionMode="None"> <CollectionView.ItemTemplate> <DataTemplate> <Frame Padding="3" CornerRadius="0"> <StackLayout Padding="10" HorizontalOptions="FillAndExpand" Orientation="Horizontal" VerticalOptions="FillAndExpand"> <Label x:Name="UserName" HorizontalOptions="FillAndExpand" Text="{Binding .}" TextColor="Black" /> <Frame Padding="7" CornerRadius="15" HeightRequest="30" HorizontalOptions="EndAndExpand" WidthRequest="30"> <Image Source="delete_user.png" /> <Frame.GestureRecognizers> <TapGestureRecognizer Command="{Binding BindingContext.DeleteUserCommand, Source={x:Reference MainPageContainer}}" CommandParameter="{Binding .}" /> </Frame.GestureRecognizers> </Frame> </StackLayout> </Frame> </DataTemplate> </CollectionView.ItemTemplate> </CollectionView> <VerticalStackLayout Grid.Row="1"> <Entry Placeholder="Type name" Text="{Binding User}" /> <Button Margin="0,10,0,0" Command="{Binding AddUserCommand}" HorizontalOptions="CenterAndExpand" Text="Add User" /> </VerticalStackLayout> </Grid> </ContentPage>
- As in the above MainPage.xaml, I have taken CollectionView to show list of user and Entry/Button to add new user.
- As you will notice, I bound the properties in the same way as we used to do it before.
MainPage.xaml.cs
namespace MAUIInvokeCommandOnListItemClickDemo; public partial class MainPage : ContentPage { public MainPage() { InitializeComponent(); BindingContext = new MainPageViewModel(); } }
Create a MainPageViewModel.cs
using CommunityToolkit.Mvvm.ComponentModel; using CommunityToolkit.Mvvm.Input; using System.Collections.ObjectModel; namespace MAUIInvokeCommandOnListItemClickDemo { public partial class MainPageViewModel : ObservableObject { [ObservableProperty] private ObservableCollection<string> userList; [ObservableProperty] private string user; public MainPageViewModel() { GetUserList(); } [RelayCommand] public void AddUser() { Application.Current.Dispatcher.Dispatch(() => { if (!string.IsNullOrEmpty(User)) { UserList.Add(User); User = string.Empty; } }); } [RelayCommand] public void DeleteUser(string user) { Application.Current.Dispatcher.Dispatch(() => { if (!string.IsNullOrEmpty(user)) { UserList.Remove(user); } }); } private void GetUserList() { UserList = new ObservableCollection<string>() { "User 1", "User 2", "User 3", "User 4", "User 5", }; } } }
5 – How to invoke command when clicking on collectionview item?
As you will see in MainPage.xaml, I have added a collection view item click event and passed the parameter to view model.
<Frame.GestureRecognizers> <TapGestureRecognizer Command="{Binding BindingContext.DeleteUserCommand, Source={x:Reference MainPageContainer}}" CommandParameter="{Binding .}" /> </Frame.GestureRecognizers>
- MainPageContainer indicates the root page.
Result
That’s all for now!
You can check the full source code here.
Happy Coding! 😀
You may also like
I got this web page from my pal who told me concerning this website and now this time I am browsing this web
page and reading very informative posts at this time.
Thanx. Good explanation!